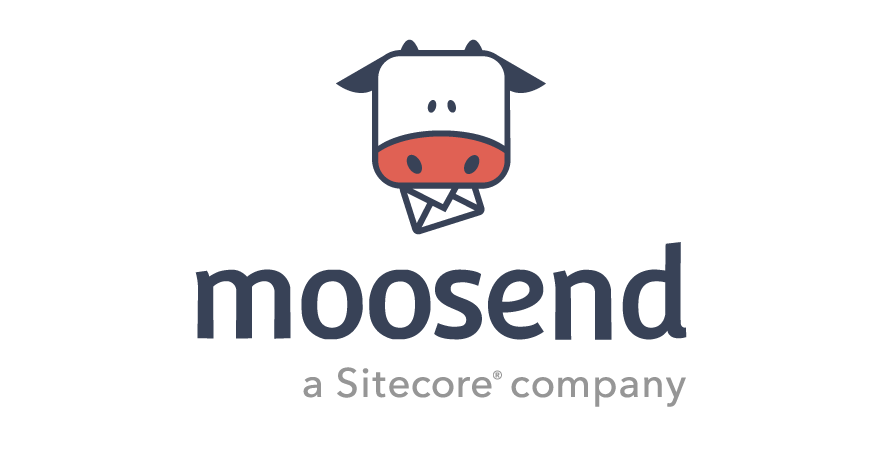
Where can I place webhooks in Sitecore Send?
Webhooks can be added to the Sitecore Send Automation as an Action.Then the URL is needed to be added:
As mentioned in the description, the endpoint should accept POST HTTP request with webhook payload.
How can I implement a custom webhook?
In this example a simple 'log' webhook will be implemented via Node.js express module.
Webhook implementation
The endpoint logic is very simple:
import express from 'express';
const app = express();
app.use(express.json());
const port = 3002;
app.post('/log', (req, res) => {
console.log(req.body, JSON.stringify(req.body));
return res.send({success: true});
});
app.listen(port, () =>
console.log(`server is listening on ${port}.`),
);
The key is app.post('/log', ...)
to handle POST request on /log
endpoint. Inside the callback we just log to console the body of request.
The full code sources can be found here.
Webhook publication
To use the webhook in Sitecore Send we need to publish it.
The following publishing options are available:
- publish it to some cloud-based platform: Azure, AWS, Google Cloud Platform, etc.
- publish local server using e.g. Ngrok
Let's use Ngrok for simplicity:
Start the server
cd [webhook folder]
npm run start
Run Ngrok
cd [ngrok location]
.\ngrok http 3002
Then the URL needs to be copied:
So the final URL for the webhook is the following:
https://3dc4-31-11-239-239.ngrok-free.app/log
Configure Webhook in Sitecore Send
Then 'Post a webhook' action needs to be added to the automation:
Explanation. The Automation 'Reactivation' is configured to be triggered when the tag 'Reactivation' is added to existing member of specified list. Then the email should be sent. So before sending the email the 'Log' webhook is added.
Results
The results of webhook work can be observed in the terminal:
The payload received from Sitecore Send:
{
"UserId":"f4deea67-ec17-4d30-854f-59cced186e59",
"StepId":"5fd8ee40-20f6-11ee-9d8a-9bf853ab0b87",
"AutomationId":"d3091761-8e4b-4d1a-8aa5-7c26bb6f5494",
"Event":{
"Id":"f288653d-8290-478f-8a94-665bc7a0bc19",
"Ab":null,
"UserId":"f4deea67-ec17-4d30-854f-59cced186e59",
"SessionId":null,
"EventName":"PROFILE_CHANGED",
"Timestamp":"2023-07-12T21:06:53.7094041Z",
"PingContext":null,
"LinkContext":null,
"BounceContext":null,
"DeviceContext":null,
"WebsiteContext":null,
"ContactContext":{
"Id":"f469d169-c38f-4717-b616-d7ebfd24194c",
"Name":"John Oliver",
"Mobile":null,
"MailingListId":"426efbad-a306-4cf2-9071-1a847285785e",
"MailingListMemberId":"f469d169-c38f-4717-b616-d7ebfd24194c",
"Tags":null,
"CustomFields":{
},
"FieldsDefinition":{
"TestFieldName":{
"Id":"c7f4bfdf-ce98-41f5-87b3-3c5cdcb5e08e",
"Name":"TestFieldName",
"Type":0,
"DateFmt":null,
"FallBackValue":"",
"IsRequired":false
}
},
"EmailAddress":"gogoh793581234@lukaat.com",
"ContactToken":"gogoh793581234@lukaat.com",
"ContactType":0
},
"LocationContext":null,
"PurchaseContext":null,
"CampaignContext":null,
"AddToCartContext":null,
"ComplaintContext":null,
"ExitIntentContext":null,
"ProductViewContext":null,
"LeadGenerationContext":null,
"ContactChangedContexts":[
{
"FieldName":"[membertags]",
"NewFieldValue":[
"reactivation"
],
"OldFieldValue":[
]
}
],
"Properties":{
"OptedInSource":"2",
"OptedInFrom":"10.201.0.252",
"OptedInOn":"2023-07-12T21:06:52.133Z",
"UpdatedOn":"2023-07-12T21:06:55.150Z",
"Tags":"[\"reactivation\"]"
}
}
}
Data scheme
Payload JSON schema can be found here.
Use cases
Webhook can be used in the following cases:
- You can setup custom notifications in Sitecore Send with a webhook.
- Data synchronization with some other storage or integration with another service.
- Logging & debugging
Links
Sitecore Send Webhooks Documentation