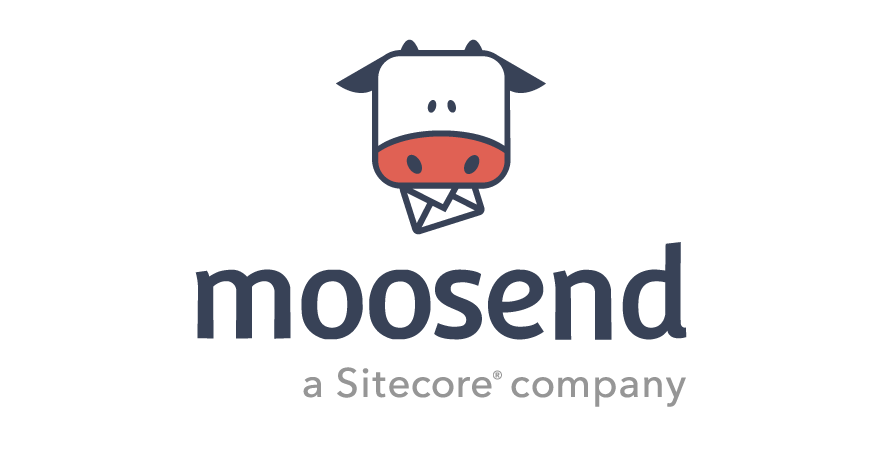
Idea behind
How Sitecore Platform can be integrated together with Sitecore Send?
Sitecore Send stores subscribers in mailing lists. So why not create create a custom submit action in Sitecore Forms to add user to mailing list? To implement it we need a way to put subscribers in the list via some API.
In this article we will review Moosend REST API, which will be used later together with Sitecore Forms integration. Also C# library usage will be described.
Moosend Mailing List API
Sitecore Send provides REST API to their services. Through the Moosend Mailing List API you can create new lists, subscribe new users to your existing lists, manage segments and create or delete custom fields. It also supports fetching list statistics such as the number of active or unsubscribed members, as well as retrieving subscriber information for a specific mailing list.
Also Moosend have C# wrapper library for that API, which can be used as Nuget package in the application. In the solution this library is used.
By default Sitecore Send API is provided as Blueprint specification, but it’s not very useful to use it for testing in a tool like Postman. So another Sitecore developer Neil Killen wrote really useful article about convert this specification to much more convenient Postman collection.
API Usage in Postman
- Obtain API key (
Account Settings
->API Key
in Moosend). See this article for more details. - Import this Postman collection to Postman.
- Set your API key in Postman:
- Start using API!
Get all mailing lists
-
Navigate to Getting all active mailing lists and fix the URL:
{{baseUrl}}/lists.{{Format}}?apikey={{apiKey}}&ShortBy=CreatedOn&SortMethod=ASC&WithStatistics=true
-
Execute request:
-
Key things in response:
{ "Code": 0, "Error": null, "Context": { "Paging": { // paging details "PageSize": 10, "CurrentPage": 1, "TotalResults": 6, "TotalPageCount": 1, "SortExpression": null, "SortIsAscending": false }, "MailingLists": [ ..., { "ID": "ab5b8e8e-8367-4efc-b08b-878ffe593805", // list id "Name": "Test", "ActiveMemberCount": 0, "BouncedMemberCount": 0, "RemovedMemberCount": 0, "UnsubscribedMemberCount": 0, "Status": 0, "CustomFieldsDefinition": [ // custom fields { "ID": "003c115b-6e9d-4a7b-8ae3-abb9056c74a9", "Name": "TestField", "Context": null, "IsRequired": false, "Type": 0, "IsHidden": false, "FallBackValue": null } ], "CreatedBy": "f4deea67ec174d30854f59cced186e59", "CreatedOn": "/Date(1659609272170)/", "UpdatedBy": null, "UpdatedOn": "/Date(-62135596800000)/" }, ... ] } }
Add subscriber to mailing list / Update subscriber in mailing list
To make this request mailing list id is required. It can be copied from previous request or from admin panel.
-
Navigate to Adding subscribers and fix the URL:
{{baseUrl}}/subscribers/:MailingListID/subscribe.{{Format}}?apikey={{apiKey}}
-
Populate path variable
MailingListID
with mailing list user is planned to be added. In our example I'll use the list namedSitecore Integration
with the following fields: -
Populate body:
{ "Name": "Paul", // subscriber name "Email": "someEmail@email.com", // subscriber email "CustomFields": [ // custom fields array, format: '{fieldName}={value}' "Is Business Account=true", "Notes=Some notes here" ], "Tags": [ // Tags specified as array "API", "Send", "Test" ] }
-
Send the request. For me the response is the following:
{ "Code": 0, "Error": null, "Context": { "ID": "ed99a263-c5c8-4533-87a6-4b0854b7a830", "Name": "Paul", "Mobile": null, "Email": "someemail@email.com", "CreatedOn": "/Date(1666821666983)/", "UpdatedOn": null, "UnsubscribedOn": null, "UnsubscribedFromID": null, "SubscribeType": 1, "SubscribeMethod": 2, "CustomFields": [ { "CustomFieldID": "aec4065a-d076-40b3-b831-e2ed99a9f0ba", "Name": "Is Business Account", "Value": "true" }, { "CustomFieldID": "94b0d4f8-19a4-4bd4-8251-f93066d81e32", "Name": "Notes", "Value": "Some notes here" } ], "RemovedOn": null, "Tags": [ "API", "Send", "Test" ] } }
-
In the Moosend subscriber should be added to mailing list:
Subscriber in list with tags:
Subscriber details:
Important. The same endpoint is used to update user information – only specified fields will be updated, if any field (like name or custom field) won’t be included in the request, then those fields will be unchanged.
C# Library
To use C# tracking library in your solution just add Nuget package Moosend.Wrappers.CSharpWrapper
to your solution.
<PackageReference Include="Moosend.Wrappers.CSharpWrapper" Version="3.0.0" />
Then to use services with DI:
public class MoosendConfigurator: IServicesConfigurator
{
public void Configure(IServiceCollection serviceCollection)
{
serviceCollection.AddScoped<IMailingListsApi, MailingListsApi>();
serviceCollection.AddScoped<ISubscribersApi, SubscribersApi>();
}
}
Get all mailing lists
const format = "json";
var apiKey = configuration.ApiKey; // your API key, obtained from settings
IMailingListsApi api = ...; // get service from DI or inject it via constructor
var response = api.GettingAllActiveMailingLists(format, apiKey);
// iterate mailing lists:
foreach (var list in response.Context.MailingLists)
{
var name = list.Name;
var id = list.ID;
var customFields = list.CustomFieldsDefinition;
}
Add subscriber / update subscriber
const format = "json";
var apiKey = configuration.ApiKey; // your API key, obtained from settings
var listId = ...; // get list id, where subscriber will be added / updated
ISubscribersApi api = ...; // get service from DI or inject it via
var customFields = new [] // populate custom fields, if needed
{
"CustomField1=Value1",
"CustomField2=Value2",
};
var request = new AddingSubscribersRequest(email, name, customFields);
// add subscriber and check success status code:
var response = api.AddingSubscribersWithHttpInfo(format, listId, apiKey, request);
var isSuccess = response.StatusCode >= 200 && response.StatusCode <= 299;
As was mentioned previously, it's pretty old package, so not all features are supported. E.g. there's no option to add Tags.
But it's possible to fix it. We need to extend AddingSubscribersRequest
with Tags
field:
public class CustomAddingSubscribersRequest : AddingSubscribersRequest
{
[JsonConstructor]
protected CustomAddingSubscribersRequest()
{
}
public CustomAddingSubscribersRequest(string email = null, string name = null, object customFields = null) :
base(email, name, customFields)
{
}
[DataMember(EmitDefaultValue = false, Name = "Tags")]
public IEnumerable<string> Tags { get; set; }
}
and then the previous example can be extended:
// as previously
var tags = new []
{
"Tag1",
"Tag2",
};
var request = new CustomAddingSubscribersRequest(email, name, customFields)
{
Tags = tags.ToList(),
};
// the rest is the same
Conclusion
Now we have enough knowledge to use Moosend REST API in our Sitecore solution. In next article we will integrate it with Sitecore Forms.