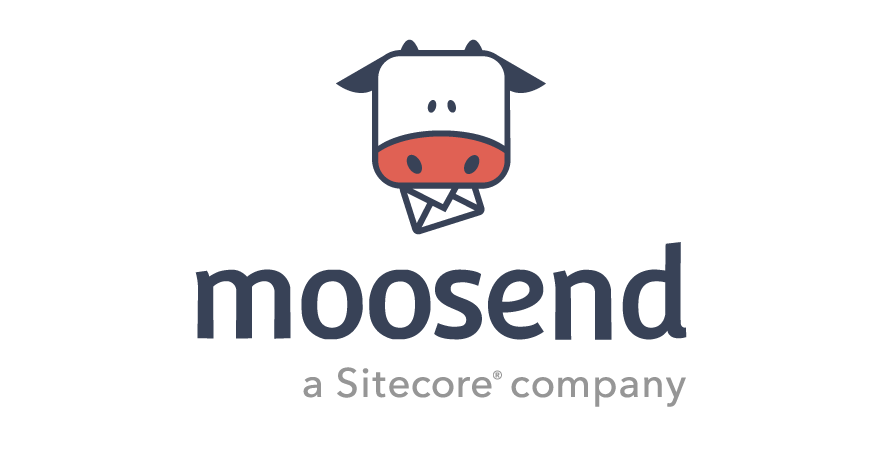
About
In previous article we reviewed Sitecore Send REST API. So now let's use Sitecore Send together with Sitecore Forms using this API. Check out my presentation on SUG Belarus for more details about this integration.Integration
How Sitecore Send and Sitecore Forms can be integrated? I see the following ways:- To send emails from Sitecore Platform to customers via Moosend SMPTP
- Create customer in Moosend after form is submitted.
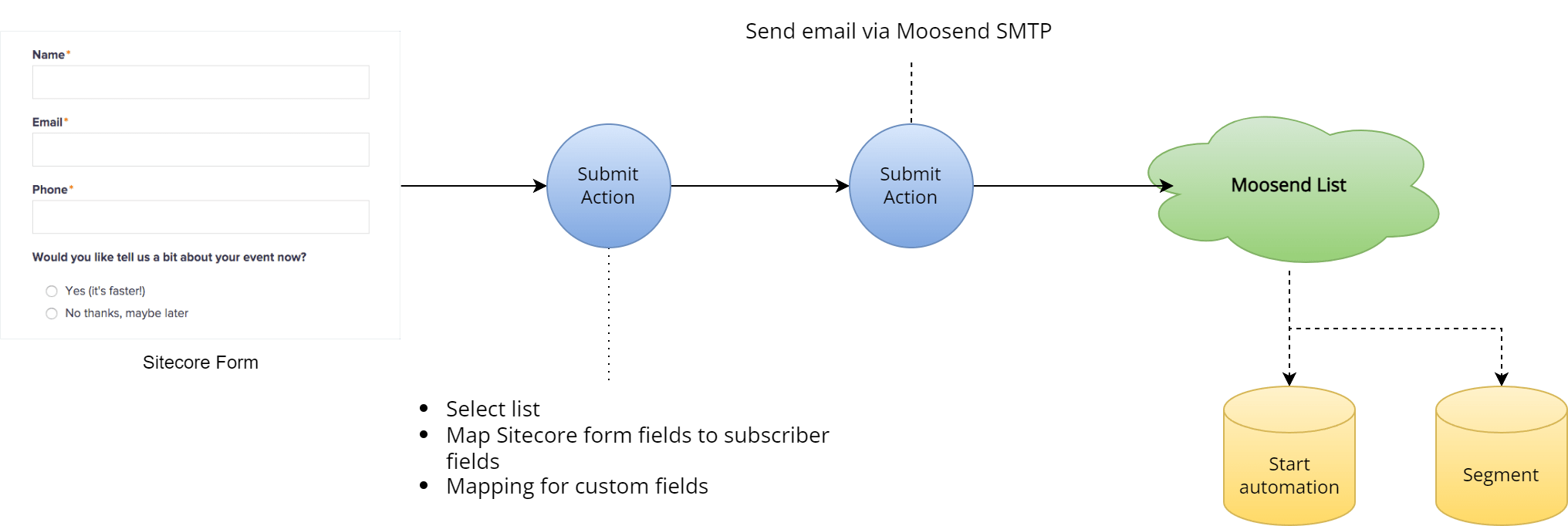
Moosend SMTP
You just need to patch Sitecore configuration with the following file:
<configuration>
<sitecore>
<settings>
<!-- Moosend SMTP settings -->
<setting name="MailServer" value="smtp.mailendo.com"/>
<setting name="MailServerUserName" value="YOU ACCOUNT EMAIL"/>
<setting name="MailServerPassword" value="YOU ACCOUNT PASSWORD"/>
<setting name="MailServerPort" value="25"/>
<setting name="MailServerUseSsl" value="false"/>
</settings>
</sitecore>
</configuration>
MailServerUserName
and MailServerPassword
are your Sitecore Send account email and password.
After patch you're able to send emails through Moosend SMTP:
using (MailMessage message = new MailMessage())
{
message.From = ...;
message.Subject = ...;
message.Body = ...;
message.IsBodyHtml = true;
message.To.Add(...);
message.CC.Add(...);
message.Bcc.Add(...);
MainUtil.SendMail(message);
}
Also OOTB with Sitecore Forms comes Send Email
submit action, which internally uses configured SMTP - so this submit action can also be used in your forms.
Add User To Mailing List
Submit Action
Submit action works in the following way.
Form builder
- User specifies the mailing list from dropdown, where subscriber should be added
- Fields mapping is configured (custom fields in Moosend to Sitecore Form fields)
See the demo:
Submit action
- Read parameters: list, fields mapping
- Map form data to model
- Add customer to mailing list via REST API
API Key
API key (Account Settings
-> API Key
in Moosend) needs to be obtained. See this article for more details.
DI registration:
serviceCollection.AddSingleton<IMoosendSettings>((pr) =>
{
var settings = pr.GetService<BaseSettings>();
return new MoosendSettings()
{
ApiKey = settings.GetSetting("Moosend.ApiKey"),
};
});
Usage (see MailingListService.cs):
public MailingListService(IMoosendSettings moosendSettings, ...)
{
_moosendSettings = moosendSettings;
...
}
public IEnumerable<MailingListJsonModel> GetAll()
{
var response =
_mailingListsApi.GettingAllActiveMailingLists(_moosendSettings.Format, _moosendSettings.ApiKey);
...
}
Submit Action Editor
- Editor structure
- JS Control
-
Service API Controller. Don't forget to register controller in DI:
serviceCollection.AddTransient<MoosendServiceApiController>();
Submit Action
Form parameters:
public class AddUserToMailingListData
{
public string List { get; set; }
public IDictionary<string, string> Mapping { get; set; } = new Dictionary<string, string>();
}
protected override bool Execute(AddUserToMailingListData data, FormSubmitContext formSubmitContext)
{
var list = data.List;
// some validation here
// get service:
var service = ServiceLocator.ServiceProvider.GetService<IMailingListService>();
// mapping:
var name = GetFieldValue(formSubmitContext, nameFieldId) as string;
var tags = GetFieldValue(formSubmitContext, tagsFieldId) as IEnumerable<string>;
var customFields = GetCustomFields(data, formSubmitContext);
// add subscriber to mailing list
var success = service.AddToMailingList(list, email, name, customFields, tags);
return success;
}
How it all works together
Check out this video (it's already with demo timecode).
Conclusion
With this integration the following features are available:
- SMTP integration
- Dynamic form and properties mapping
All sources are available on my Git Repo.