Sitecore Search and Ingestion API
Sitecore Search serves as a headless content discovery platform, empowering users to craft predictive and personalized search experiences spanning various content sources Sitecore Search | Sitecore Documentation. In this article, I aim to share my firsthand experience with Sitecore search and Ingestion API Ingestion API | Sitecore Documentation, detailing the process of creating a data source, pushing data to the source, as well as entities and widgets.
The initial step in commencing work with Sitecore search involves the creation of a new data source. The developer should navigate to the "Sources" section in the menu and subsequently click on the "Add source" button.
Within the new window, it is essential to configure the source name, description, and connector. The available connector options include Api Crawler, Api Push, Feed Crawler, Web Crawler, and Web Crawler (Advanced). Given that this article focuses on utilizing the ingestion API, it is imperative to select "Api Push" as the connector for the source.
After saving, you will see a new source on the previous page "Sources".
Upon accessing the newly created source, essential information required for pushing data to this source will be available. The pertinent information necessary for pushing data to this source comprises the "Source ID," "Domain ID," "Endpoint URL," and "API Key."
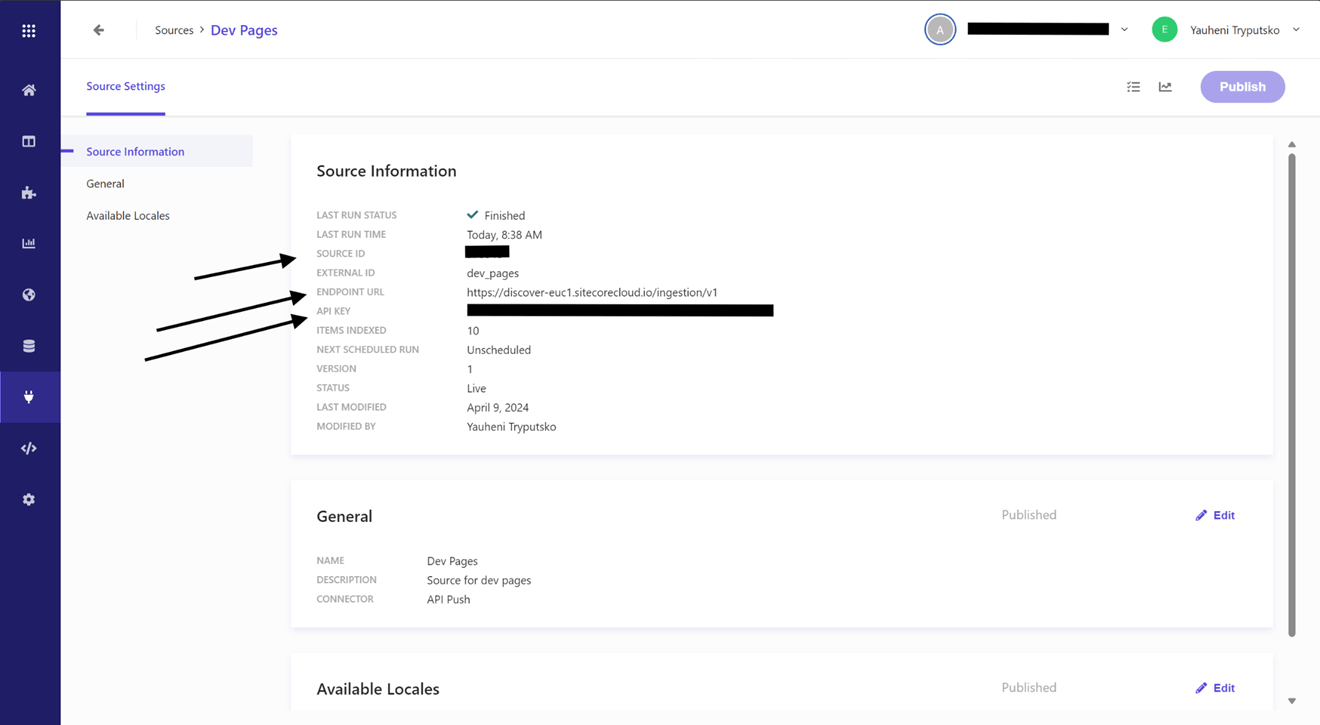
The Domain ID is typically included in the URL as a parameter, specifically as "?domain=". Currently, the data can only be pushed with the entity type set as "content," as it serves as the default entity in Sitecore Search. Example code for pushing data:
public static async Task SendDataToSitecoreSearch(int id)
{
try
{
string apiUrl = "https://discover-euc1.sitecorecloud.io/ingestion/v1/domains/{your domain id}/sources/{your source id}/entities/content/documents?locale=en_us";
string jsonBody = @"
{
""document"": {
""id"": ""id_value"",
""fields"": {
""name"": ""Example page id_value"",
""description"": ""This is test page id_value"",
""type"": ""content page"",
""url"": ""test.com/page-id_value"",
""tags"": [""content"", ""main""]
}
}
}";
jsonBody = jsonBody.Replace("id_value", id.ToString());
HttpClient client = new HttpClient();
// Adding Authorization header
client.DefaultRequestHeaders.Add("Authorization", "your api key");
// Content
var content = new StringContent(jsonBody, Encoding.UTF8, "application/json");
// Send POST request
HttpResponseMessage response = await client.PostAsync(apiUrl, content);
// Check response status
if (response.IsSuccessStatusCode)
{
string responseContent = await response.Content.ReadAsStringAsync();
Console.WriteLine("Request sent successfully. Response:");
Console.WriteLine(responseContent);
}
else
{
Console.WriteLine("Request failed with status code " + response.StatusCode);
}
}
catch (Exception ex)
{
Console.WriteLine("An error occurred: " + ex.Message);
}
}
After submitting the POST request, you can monitor the progress of your request by accessing the following location:
The Ingestion API offers four distinct methods for processing data:
1. Create Document: This method facilitates the creation of a new document within the search index.
2. Update Document: With this method, existing documents in the search index can be updated with new information or modifications.
3. Delete Document: It enables the removal of specified documents from the search index.
4. Partial Update Document: This method allows for making partial updates to existing documents in the search index without having to resend the entire document.
You can find detailed information on each of these methods in the documentation provided Ingestion API | Sitecore Documentation.
Indeed, the Ingestion API is straightforward and consistent in its usage. For the update, delete, and partial update methods, the only additional requirement is to include the "id" parameter in the URL to specify the document to be updated, deleted, or partially updated. This maintains simplicity and uniformity across the various operations offered by the API.
Now you can work with the data, but only with a standard entity as the content. This entity has fields and looks as shown in the figure below:
You have the ability to generate custom entities featuring custom list fields. However, in this article, I won't delve into this process. Instead, I'll reserve it for future articles where I'll demonstrate how to effectively utilize custom entities. Additionally, to retrieve data for search purposes, you'll need to create a widget or use default widgets.
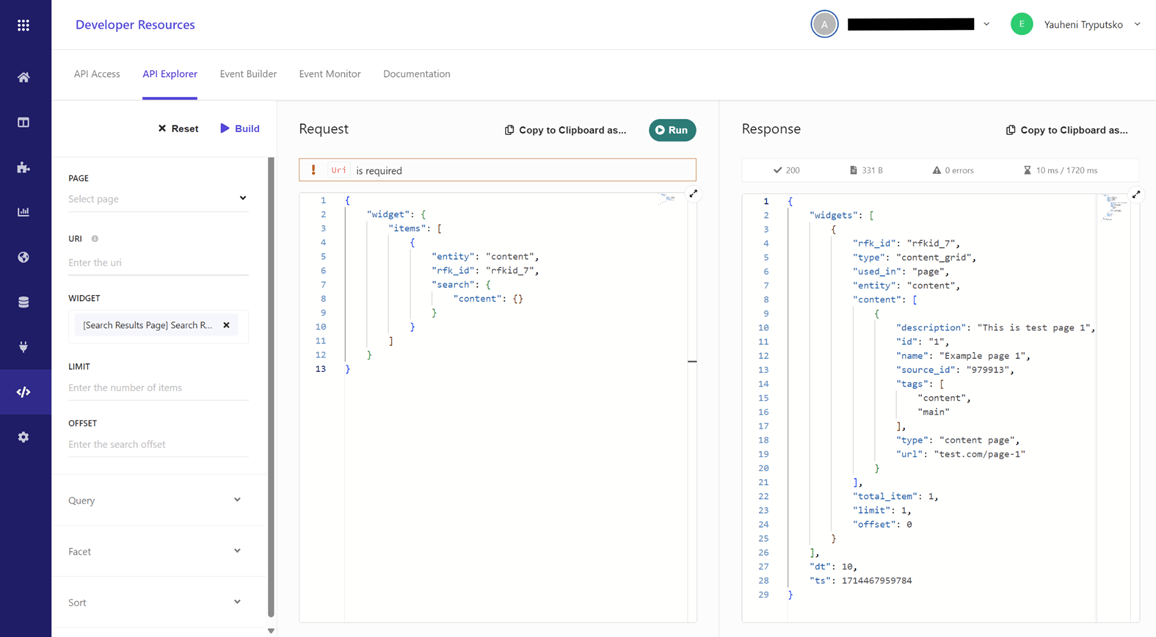