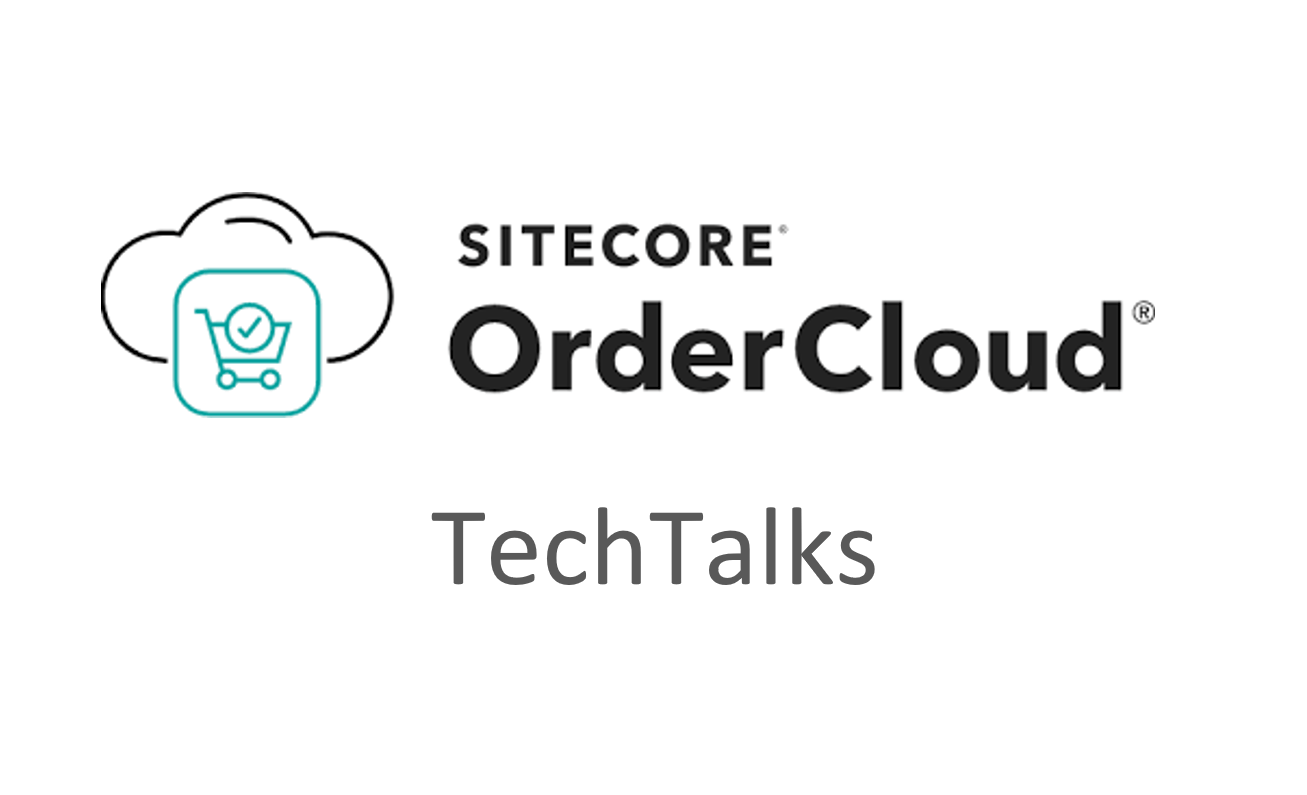
Working on Sitecore projects hosted in Docker or in Kubernetes, sometimes we need to add custom code or config changes to the Identity server, XConnect, Marketing Automation, etc. This article describes the approach to achieve that, which is common and applies to all Sitecore roles.
During the last project, which is a corporate website, I had the need to add a config with a custom Client configuration to the Identity server. This client configuration allows you to log in to Sitecore through Sitecore CLI using a secret token. That is useful when you need to build CI/CD processes and you need to run serialization/publish/index rebuild remotely. So that is a good example to explore.
First of all, we need to create a Class Library project within our solution that will contain our custom, identity server-related, changes. I have called this project Corporate.Environment.Identity
All custom configs need to be placed in the Config folder. I have added only one file Sitecore.IdentityServer.Corporate.xml with the following content:
<?xml version="1.0" encoding="utf-8"?> |
The ClientId and ClientSecret1 will be used to log in to Sitecore during the CI/CD process.
Since we don’t need anything else, we are ready to switch to updating our docker images.
First of all, we need to add a build command in our solution Dockerfile and copy build artifacts to the image:
# escape=` |
Once our solution image contains the required artifacts, we need to update our docker-compose.override.yml to pass the solution image to the identity server build:
# Use our retagged Identity Server image. |
Then we need to update the identity server build docker file itself to copy config from solution image to the identity one:
|
After the next docker-compose build all new configs will be deployed to the docker. It allows us to use Sitecore CLI within CI/CD processes. You can find the Powershell script to run Sitecore Serialization sync below:
function Arg { param([Parameter()][string]$Parameter) return $Parameter } |