In one project, a customer wanted to create pages with video components to show teasers, clips, and other video types to the users. Our team investigated how we could simply implement this task and we found Brightcove.
TODO: info about Brightcove https://www.brightcove.com/
//info from sitecore, maybe need to changes
Brightcove, the global leader in video for business, is a powerful cloud-based solution for publishing, distributing, measuring, and monetizing broadcast-quality video across devices. Since 2004, Brightcove has built the world's most intelligent video streaming and communications platform – offering limitless scale, world-class security, and powerful integrations to help companies:
Monetize Content. Customers love the flexible layouts, monetization options, and unmatched reliability our powerful streaming platform delivers. Ad-based, subscription-based, or hybrid...run ads before, during, or after content...with server-side ad insertion (SSAI), the model to use is completely up to them.
Engage Audiences. Put people’s favorite form of communication to work. Video lets customers reach their audiences wherever they are, connecting through live virtual events, and training teams spread across the world. With the security customers need, reliability they can trust, and impact they can measure, Brightcove video is a no-brainer for creating global connections.
Sell Products. Video has the power to turbocharge marketing plans. Whether customers are looking to generate more leads, drive brand awareness, bring their products to life, livestream events, or reach a global audience, there’s no better way to connect than video.
In brief, Brightcove enables customers to quickly, easily, and securely distribute broadcast-quality video to Internet-connected devices globally - launching faster and scaling instantly. With award-winning technology and customer support, Brightcove helps businesses in more than 70 countries inspire, entertain, and educate. Customers rely on our suite of products, services, and expertise to reduce the cost and complexity associated with video.
Why Brightcove:
- a good user interface for uploading videos
- tools for adding subtitles, teaser images, etc.
- information about views
- simple integration with Sitecore
For the integration of Brightcove into Sitecore we need to follow some easy steps:
- To install Brightcove.Media.Framework-10.0.zip (install package through Sitecore Installation Wizard)
- To get Account Credentials from Brightcove and set up them into Sitecore
- To upload video through Brightcove web interface and import data to Sitecore
You can find more information at https://integrations.support.brightcove.com/sitecore/getting-started-brightcove-video-connect-sitecore-experience-platform.html#contents
Sitecore video components using SitecoreJSS, GraphQL, and Brightcove
You can read about what Brightcove is here “Link on above information” or at https://www.brightcove.com/.
After installing the package, you should get the items for setting up Brightcove, video information items, and a menu section with buttons for importing/exporting video items, cleanup, etc.
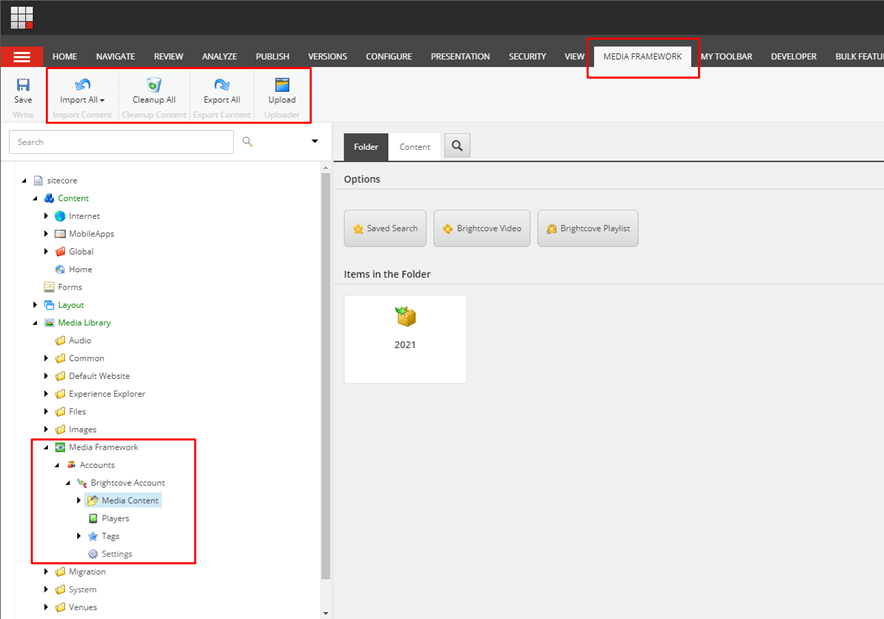
For correct work of Brightcove with Sitecore, you should have settings “Publisher ID”, “Client Id”, “Client Secret”.
All media content will be created in Media Content. Media Content has a bucket structure.
Media items have “/sitecore/templates/MediaFramework/Brightcove/BrightcoveVideo” templates, you should get all templates after installing the Media Framework package for Brightcove. This template has fields with information about video: ID, Name, Created Date, Description, Tags, etc.
For my project, I use SitecoreJSS and GraphQL technology for implementation api. Of course, you can work with Brightcove Video items from Media Content, but sometimes you should have additional information that you don’t have in Brightcove. Example: Title, Preview Images (from Sitecore). For that, you need to create a custom template where you will have a link to a video item from Media Content.
If you use GraphQL for your api, you need to create Json Rendering (/sitecore/templates/Foundation/JavaScript Services/Json Rendering) and implement the “ComponentQuery” field. Example GraphQL as shown below:
query IntegratedQuery($contextItem: String!) {
contextItem: item(path: $contextItem) {
... on VideoDetailsPage {
name
title {
jss
}
introText {
jss
}
video {
jss
}
}
}
videoSetting: item(path: "/sitecore/media library/Media Framework/accounts/Brightcove Account") {
... on BrightcoveAccount {
publisherID {
jss
}
}
}
}
Title and Intro text are custom fields that I created in the “Video Page” template. Video field is a link field on Video Item from Media Contents. Also for outputting video you need to transfer the information as Publisher ID from Brightcove Account Setting.
I implemented the front-end part on ReactJs. This is an example of how to output a video:
import React from "react";
import ReactPlayerLoader from '@brightcove/react-player-loader';
export interface VideoItem {
title: JssField<string>,
introText: JssField<string>
video: {
jss: Video
}
}
export class BrightcoveVideoPlayer extends React.Component<VideoItem> {
render() {
return (
ReactDOM.createPortal(
<ControllPanel
nextPrevClick={(isNext: boolean) => {
player.trigger('rewind', { 'amount': rewindTime, 'isNext': isNext })
}}
playPauseClick={(value: boolean) => {
this.playPauseFunction(player, value)
}}
isPlay={!player.paused()}
player = {player}
setVolume = {this.setVolume}
volume = {this.state.volume}
/>,
spacer
)
<ReactPlayerLoader
className={commonStyles}
accountId={this.props.accountId}
videoId={this.props.videoId}
options={{
autoplay: this.props.autoPlay,
loop: this.props.loop,
muted: this.props.muted
}}
onSuccess={(success: any) => {
var myPlayer = success.ref;
const _self = this;
this.addRewindClick(myPlayer)
this.changeVideoDuration(myPlayer)
setVideoTimestamp && this.changeVideoTimestamp(myPlayer)
myPlayer.on('pause',function() {
})
myPlayer.on('play',function() {
})
myPlayer.on('loadedmetadata', function () {
})
myPlayer.on('ended',function(){
});
}}
/>
)
}
}
The main parameters are accountId (it is Publisher ID from Sitecore) and videoId.
ReactJs without JSS context:
<!-- for testing, use the unpkg.com files -->
<script crossorigin src="https://unpkg.com/react@16/umd/react.development.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@16/umd/react-dom.development.js"></script>
<script src="https://solutions.brightcove.com/bcls/brightcove-player/reactjs/brightcove-react-player-loader.min.js"></script>
<h2>React sample using script tags</h2>
<div id='container'></div>
var React = window.React;
var ReactDOM = window.ReactDOM;
var ReactPlayerLoader = window.BrightcoveReactPlayerLoader;
// +++ Add the Brightcove Player +++
var reactPlayerLoader = window.reactPlayerLoader = ReactDOM.render(
React.createElement(ReactPlayerLoader, {
accountId: '1752604059001',
videoId: '5819230967001',
onSuccess: function(success) {
// two ways to get the underlying player/iframe at this point.
console.log(success.ref)
console.log(reactPlayerLoader.player);
}
}),
document.getElementById('container')
);